반응형
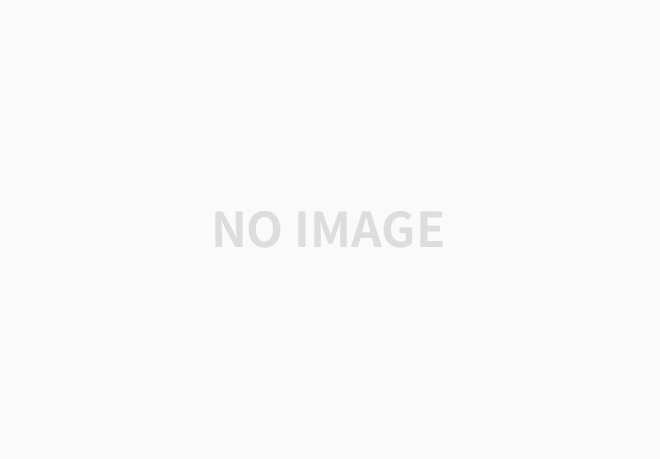
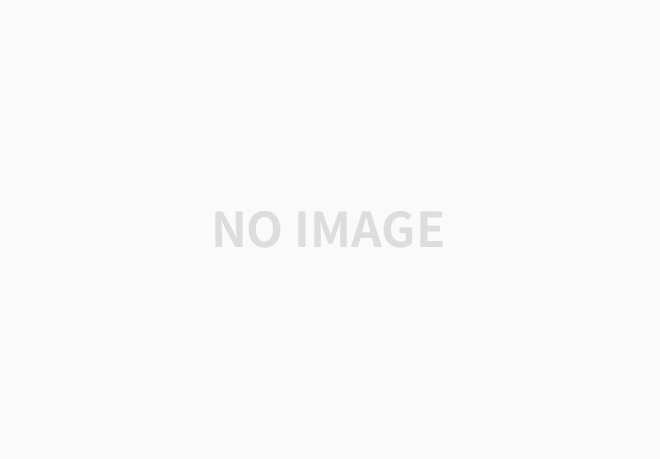
977. Squares of a Sorted Array
Given an integer array nums sorted in non-decreasing order, return an array of the squares of each number sorted in non-decreasing order.
Example 1:
Input: nums = [-4,-1,0,3,10]
Output: [0,1,9,16,100]
Explanation: After squaring, the array becomes [16,1,0,9,100].
After sorting, it becomes [0,1,9,16,100].
Example 2:
Input: nums = [-7,-3,2,3,11]
Output: [4,9,9,49,121]
Constraints:
- 1 <= nums.length <= 104
- -104 <= nums[i] <= 104
- nums is sorted in non-decreasing order.
📖 문제. 해당 배열의 제곱값을 오름차순으로 정렬하시오.
class Solution {
public int[] sortedSquares(int[] nums) {
for(int i=0; i<nums.length; i++){
nums[i] *= nums[i];
}
Arrays.sort(nums);
return nums;
}
}
JAVA SOLUTION CODE 👍
class Solution {
public int[] sortedSquares(int[] A) {
int n = A.length;
int[] result = new int[n];
//we have positive end of the numberline and negative end
//which ever end's absolute value is larger then it is the bigger one
int i = 0, j = n - 1;
for (int p = n - 1; p >= 0; p--) {
if (Math.abs(A[i]) > Math.abs(A[j])) {
result[p] = A[i] * A[i];
i++;
} else {
result[p] = A[j] * A[j];
j--;
}
}
return result;
}
}
189. Rotate Array
Given an array, rotate the array to the right by k steps, where k is non-negative.
Example 1:
Input: nums = [1,2,3,4,5,6,7], k = 3
Output: [5,6,7,1,2,3,4]
Explanation:
rotate 1 steps to the right: [7,1,2,3,4,5,6]
rotate 2 steps to the right: [6,7,1,2,3,4,5]
rotate 3 steps to the right: [5,6,7,1,2,3,4]
Example 2:
Input: nums = [-1,-100,3,99], k = 2
Output: [3,99,-1,-100]
Explanation:
rotate 1 steps to the right: [99,-1,-100,3]
rotate 2 steps to the right: [3,99,-1,-100]
Constraints:
- 1 <= nums.length <= 105
- -231 <= nums[i] <= 231 - 1
- 0 <= k <= 105
📖 문제. 배열을 오른쪽으로 k단계씩 회전하시오.
class Solution {
public void rotate(int[] nums, int k) {
int[] answer = new int[nums.length];
for(int i=0; i<nums.length; i++){
answer[(i+k)%nums.length] = nums[i];
}
for(int i=0; i<nums.length; i++){
nums[i] = answer[i];
}
}
}
300x250
'Algorithm > LeetCode' 카테고리의 다른 글
[LeetCode] Algorithm I - Day 4 Two Pointers (0) | 2022.10.28 |
---|---|
[LeetCode] Algorithm I - Day 3 Two Pointers (0) | 2022.10.27 |
[LeetCode] Algorithm I - Day 1 Binary Search (0) | 2022.10.26 |
[LeetCode] 371. Sum of Two Integers (두 숫자의 합) (1) | 2022.05.24 |
[LeetCode] 35. Search Insert Position (0) | 2022.04.20 |